As part of my course work for Design Studio (LDT550) at Penn State, I am learning how to program in Swift (Apple’s iOS programming language) using xCode 6 beta. Â In particular, I am working with Dr. Simon Hooper to get some of the basics down by programming a simple calculator.
Here is my progress…
First of all, connecting UI elements from storyboard (Main.storyboard) to the code that controls (ViewController.swift) isn’t too difficult. Â It’s a bit abstract and there are multiple ways to connect them (ctrl+drag from storyboard or using the connections inspector), but once you figure it out, it seems straightforward.
The actual programming was more challenging than I expected.  I never thought about the intricacies of calculators.  Modern devices have changed our expectations on how calculators work.  Our current expectations are closer to how graphing calculators operate.
For example, in an basic calculator, you can enter a number and as soon as you press an operator, it clears the screen and expects the next number:
Press 1 (display shows “1”)
Press + (display shows “+” and clears old value waiting for new value)
Press 1 (display shows “1”)
Press = (display shows “2”)
However, in a graphing calculator, you can enter an entire expression before calculating
Press 1 (display shows “1”)
Press + (display shows “1 +”)
Press 1 (display shows “1+1”)
Press = (display shows “2” or “1+1=2”)
There are many design decisions to make before programming even a simple calculator – and the design decisions are not simply visual – not by a long shot.
Some technical lessons learned:
- Type inference and type safety came into play right away (https://developer.apple.com/library/prerelease/mac/documentation/Swift/Conceptual/Swift_Programming_Language/TheBasics.html).
- Converting to and from Strings is a bit tricky (for display onto a Text Field) – I may not be doing it correctly right now – need to learn some more about this.
- Syntax is very familiar, but it’s unnatural not to end lines with a semi colon.
- xCode hints and autocomplete feature is very helpful and educational.
- I am not quite sure how the layout works (with the square View Controller – which I assume it’s for designing apps that will work well for landscape/portrait/different size devices), so I have placed everything close to the top/left to start.
Here’s the app so far (only + works, not thoroughly tested):
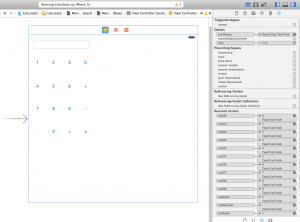
And here’s the code:
// // ViewController.swift // Calculator // // Created by Jin S. An on 10/1/14. // Copyright (c) 2014 jinsungpsu.com. All rights reserved. // import UIKit class ViewController: UIViewController { var displayString = "" @IBOutlet var calcDisplay: UITextField! override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view, typically from a nib. } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() // Dispose of any resources that can be recreated. } func updateCalculatorDisplay(buttonPressed: String) { displayString += buttonPressed + " " calcDisplay.text = displayString } @IBAction func calc01(sender: AnyObject) { updateCalculatorDisplay("1") } @IBAction func calc02(sender: AnyObject) { updateCalculatorDisplay("2") } @IBAction func calc03(sender: AnyObject) { updateCalculatorDisplay("3") } @IBAction func calc04(sender: AnyObject) { updateCalculatorDisplay("4") } @IBAction func calc05(sender: AnyObject) { updateCalculatorDisplay("5") } @IBAction func calc06(sender: AnyObject) { updateCalculatorDisplay("6") } @IBAction func calc07(sender: AnyObject) { updateCalculatorDisplay("7") } @IBAction func calc08(sender: AnyObject) { updateCalculatorDisplay("8") } @IBAction func calc09(sender: AnyObject) { updateCalculatorDisplay("9") } @IBAction func calcDecimal(sender: AnyObject) { updateCalculatorDisplay(".") } @IBAction func calc00(sender: AnyObject) { updateCalculatorDisplay("0") } @IBAction func calcPlus(sender: AnyObject) { updateCalculatorDisplay("+") } @IBAction func calcMinus(sender: AnyObject) { } @IBAction func calcMultiply(sender: AnyObject) { } @IBAction func calcEqual(sender: AnyObject) { var tempArray = displayString.componentsSeparatedByString(" ") var currentOperation = "" var calculatedValue:Float = 0.0; for tempValue in tempArray { displayString += tempValue if tempValue == "+" { currentOperation = "+" } else if currentOperation == "" { calculatedValue = (tempValue as NSString).floatValue } else { if currentOperation == "+" { calculatedValue += (tempValue as NSString).floatValue } } } displayString = "=" + calculatedValue.description calcDisplay.text = displayString } @IBAction func calcClear(sender: AnyObject) { displayString = "" calcDisplay.text = "" } }